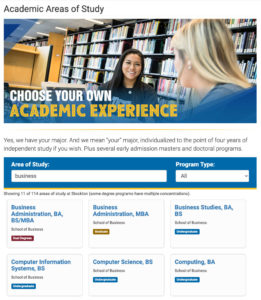
We made a pretty slick degree chooser at work. Ours is labeled as Areas of Study, but ultimately we made an easier way for our visitors to sift through what degree(s) might appeal to them.
Live example:
https://stockton.edu/academic-affairs/academic-schools-programs.html
There’s a lot going on under the hood with this one, and we’ve utilized what I think are some pretty cool techniques to make it not only easy to use but also easy for our content managers to keep up to date.
Our CMS (content management system) is OU Campus which specializes in higher ed. They’re great for us. Their templating system relies on XML/XSLT (not always so great), so ultimately a content manager typically is editing an XML file on the staging server that is then transformed into an HTML file that live on the production server for visitors to see.
So to make editing as seamless as possible, we setup a table is OU Campus that our content manager can edit, just like any regular page. Just what they’re comfortable doing day in and day out. We then leverage some XSLT magic (there are parts of it that I still believe to be magic even though I wrote it) to transform the content managers edits. Here’s what’s happening when they edit:
- Content manager makes normal edits to a html table with columns, rows, etc. Each degree is a new row in the table.
- On publish the XSLT transforms the edited XML into 2 files. A standard HTML page of the table transform and grouped by degree type.
- This is the most basic but functional version of the page. It’s served up like this to meet any visitors who may be visiting with the most ancient of browsers. They will still at minimum get a fully functioning list of linked degrees.
- The table is also transformed via another XSLT file to a JSON file. This JSON file will serve as our data source of areas of study. The external JSON file makes it easier to use the data in other pages/applications too!
- So now we have 2 files. The HTML page which is fully functional, yet a tad boring and a JSON file just waiting to be used.
Next we used Vue to write the JavaScript for this little app. I found Vue to be pretty lightweight and translates well to our CMS and the talents of our team. It’s my current favorite way to code up JS projects.
We didn’t use the vue-cli, just some vanilla Vue for this one. If we do a 2.0 we’ll probably go with vue-cli as we have a much better understanding of it now and it’s benefits.
Vue
4. The Vue script not only filters by title, school, etc. but we also include a field for tags. This really opens the door for us to make sure that our degrees can be found not only on their proper name, but perhaps a name that is used at other institutions, or even if searched as a career driven term. This should be a big upgrade for all.
5. To top it off we collect Events in Google Analytics of terms searched on. This data will help us make sure that the tags being used make sense and if we should consider adding new tags or even renaming or launching new degrees in the future.
All in all a very fun project that really helps to modernize a highly used page on our site. We’re looking to incorporate the JSON data into other apps/uses as well.
Here’s the repository on GitHub: